Today, JavaScript is widely used. It works with both your frontend and your backend. Furthermore, the JavaScript environment is heavily reliant on third-party libraries. As a result, protecting JavaScript necessitates adhering to best practices in order to reduce the attack surface.
But how do we ensure that JavaScript apps are safe?Let's have a look.
1. JavaScript Integrity Checks
You may have used <script> tags to import third-party libraries as a frontend developer. Have you considered the security implications of doing so?
a. What happens if a third-party resource is tampered with?
Yes, when you make external services on your web, these things will happen.
As a consequence, the website could be vulnerable to hackers.
As a precaution, you should use the following code in your script: honesty (also known as Subresource integrity — SRI).
<script
src="https://code.jquery.com/jquery-3.3.1.slim.min.js"
integrity="sha384-q8i/X+965DzO0rT7abK41JStQIAqVgRVzpbzo5smXKp4YfRvH+8abtTE1Pi6jizo"
crossorigin="anonymous">
</script>
If the source has been tampered with, the integrity attribute allows a browser to search the fetched script to guarantee that the code is never loaded.
If the source has been tampered with, the integrity attribute allows a browser to search the fetched script to guarantee that the code is never loaded.
b. Frequent Tests for NPM Vulnerabilities
I'm sure you're both aware that the npm audit command may be used to find bugs in all installed dependencies. It generates vulnerability reports and recommends repairs. But can you do so on a regular basis?
These bugs will pile up until we simplify it, making it impossible to repair them.
Remember that some of them can be crucial, allowing for serious exploits.
To find bugs, you should run NPM in your CI for each pull request as a solution.
As a result, you can avoid any bugs going undetected.
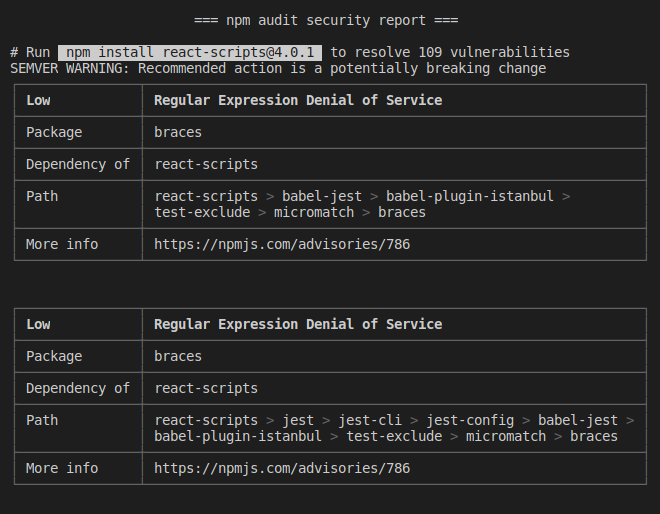
Any vulnerabilities, on the other hand, would necessitate manual intervention by a developer.
c. Extra from Github
Recently, GitHub released Dependabot, a bot that automatically scans NPM dependencies and sends you an email with the threats.
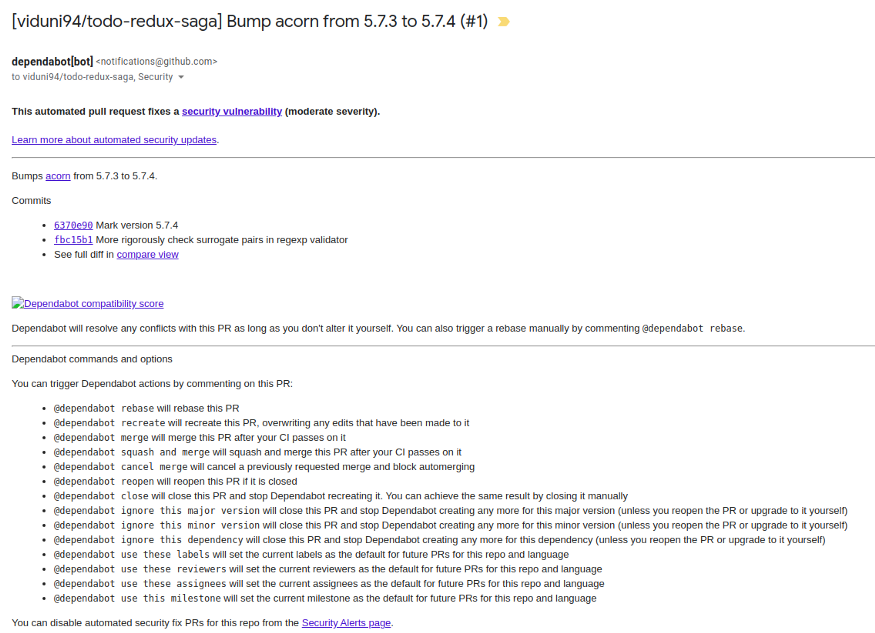
Furthermore, suppose you allowed the choice for "automated protection patch PRs." In any case, GitHub would automatically submit a PR to correct the bugs, resolving the security problems ahead of time.
d. Build & share independent components with Bit
Bit is a highly extensible tool that allows you to build fully scalable applications with modules that are separately written, versioned, and maintained.
It can be used to create modular apps and design frameworks, as well as author and distribute micro frontends and simply share components between apps.
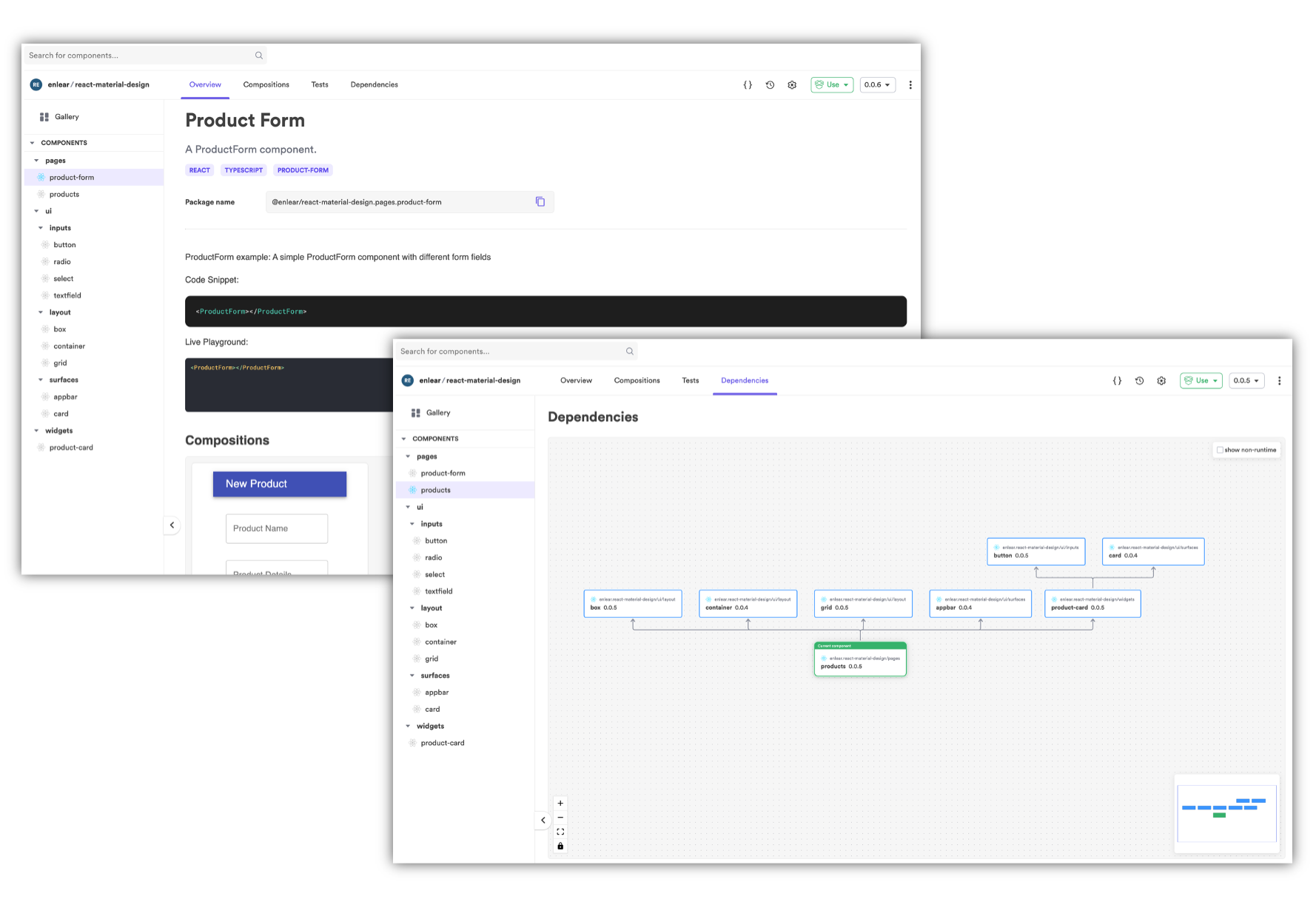
2. Keep Minor and Patch Version Updates Enabled
Have you ever seen ^ or ~ in front of a variant of an NPM package? For small and patch variants, these symbols denote an automatic update bump (depending on the symbol).
Minor and fix variants are backward compliant in terms of technology, lowering the possibility of adding glitches into the program.
Since most third-party libraries issue hot-fixes as patch version bumps, allowing automatic patch releases at the very least helps to minimize security risks.
3. Have Validations in Place to Avoid Injections
As a general rule, we can never depend solely on client-side validations because attackers can alter them whenever they want. However, by providing validations for each input, certain JavaScript injections can be avoided.
If you enter something in the comment field with quotes — <script><script/>
, for example, those quotes will be replaced with double — <<script>><</script>>
. The JavaScript code you entered will not be executed. Cross-Site Scripting is the term for this (XSS).
There are a few more popular methods for injecting JavaScript as well.
- To attach or modify JavaScript, use the developer's console.
- In the address bar, type "javascript:SCRIPT."
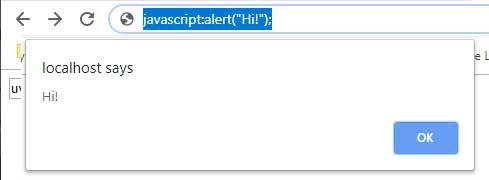
To keep your application safe, you must prevent JS injections. As I previously said, getting validations in order is one way to avoid it. Replace all <
with <
, and all >
with >
.
4. Content Security Policies
Another way to prevent malicious injections is to use Content Security Policies (CSP). The following steps will show you how to use CSP.
Content-Security-Policy: trusted-types;
Content-Security-Policy: trusted-types 'none';
Content-Security-Policy: trusted-types <policyName>;
Content-Security-Policy: trusted-types <policyName> <policyName> 'allow-duplicates';
For more information about CSPs refer to these guidelines.
5. Always Keep Strict Mode On
When Strict mode is enabled, you will be prevented from writing unsafe code.
Furthermore, enabling this mode is easy. It's as simple as placing the following line at the top of your JavaScript files.
use strict
When strict mode is on;
- Errors are thrown on any errors that were previously ignored.
- Corrects errors that make it impossible for JavaScript engines to optimize.
- The usage of reserved terms that are likely to be specified in future versions of ECMAScript is prohibited.
- When ‘unsafe' acts are made, mistakes are thrown (such as gaining access to the global object).
For years, strict mode has been supported by any modern browser. The word is simply overlooked if the browser does not allow strict mode.
6. Lint Your Code
Linters analyze the codebase from a static perspective. It aids in the establishment of consistency and the avoidance of traditional pitfalls. Linting aims to reduce security vulnerabilities and consistency and security go hand in hand. The following are some of the most common JavaScript tools that we use.
- JSLint
- JSHint
- ESLint
Additionally, software like SonarCloud can be used to detect code smells and suspected security flaws. The following is an example of a Sonar report.
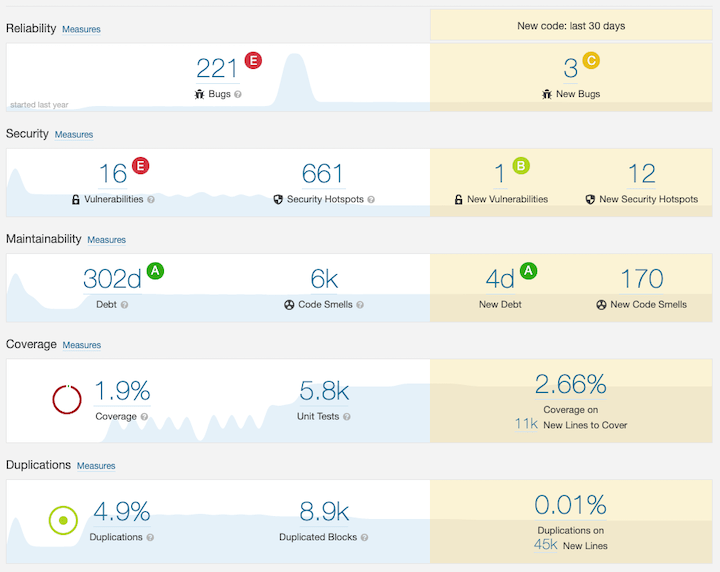
Note: As you can see in the picture above, there is a protection section that displays bugs and security hotspots.
7. Reduce the size of the code by minifying and uglifying it
Attackers will almost always attempt to decipher the code in order to gain access.
As a result, using readable source code in the production construct expands the attack surface.
It is standard practice to minify and uglyfy JavaScript code in order to make exploiting bugs in the code more challenging.
If you want and take extra precautions to keep the code hidden from users/clients, keep it on the server side and don't submit it to the browser at all.
Summary
To make your application safe, it's critical to focus on security, particularly in JavaScript applications. JavaScript can be protected with only a few resources to deter common attacks.
Furthermore, let's say you're looking for advanced options. In any case, there are tools like Snyk and WhiteSource that specialize in scanning the code for bugs and automating the process with continuous integrations.